# Step-by-step guide for getting up and running with Ruby and Informix
God forbid that anyone ever needs to use an Informix database with their Ruby on Rails application. In you case you do, here's how I did it for getting up and running with Ruby and Informix. In my case I was not using Informix as my main database, but did need to pull in information from multiple databases setup on Informix, hence the reason for digging in and figuring out how to get everything to cooperate.
# 1. Download and install Infromix Client SDK
Depending on what kind of system you're running your application on you'll want to download the appropriate package from https://www-01.ibm.com/marketing/iwm/tnd/search.jsp?rs=ifxdl. In our case we're using a linux 64bit environment. Once extracted, the package contains a file called installclientsdk
. Simply run the file with administrative permissions like so sudo ./installclientsdk
and follow the appropriate steps.
The default directory install is /opt/IBM/informix
. You'll want to set a required environment variable called INFORMIXDIR
which corresponds to the install directory for the SDK.
# in ~/.bash_profile for any users that need access
export INFORMIXDIR=/opt/IBM/informix # or appropriate directory
# 2. Create sqlhosts file and add configuration
The sqlhosts file contains the configuration for any database hosts that you would like to connect to. The Client SDK comes with a sample sqlhosts file as shown below.
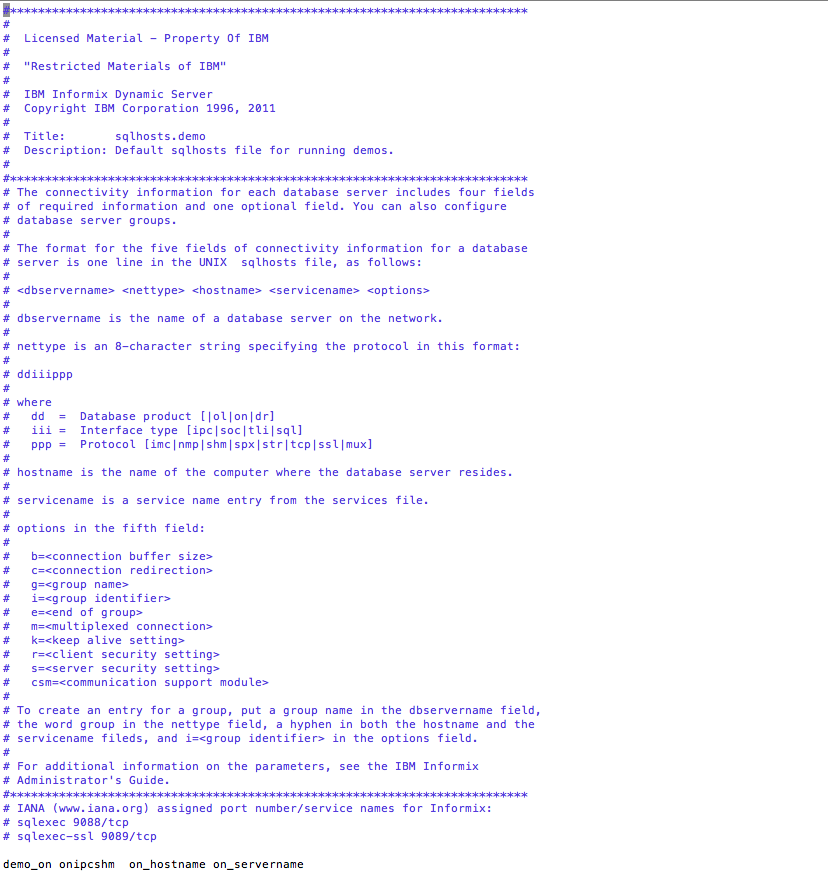
Create a new file with the following pathname $INFORMIXDIR/etc/sqlhosts
and add the appropriate configuration.
# Explanation of Variables
- <dbservername> - this will be the name that the Ruby client will use to get the right info for the database
- <nettype> - protocols as explained in image. For our case we will use onsoctcp
- <hostname> - hostname or ip address
- <servicename> - service name as described by the database's /etc/services file. Can also just use the port number.
# 3. Create Environment variables for any user who needs access
The following environment variable must be added for any user who needs access (on top of $INFORMIXDIR
)
# ~/.bash_profile
# Choose a default database server name as defined in $INFORMIXDIR/etc/sqlhosts
export INFORMIXSERVER=dbservername
# 4. Install ActiveRecord adapter for informix
An activerecord adapter for informix can be found at https://github.com/santana/activerecord-informix-adapter. Add the gem to your Gemfile and run bundle install. The active record adapter depends on the informix gem which can be used for plain ruby applications
# Gemfile
gem 'activerecord-informix-adapter'
At this point, the gem should install properly as long as everything else was configured properly.
# 5. Connecting to the database with Ruby/Rails
You should now be able to connect to the database using the establish_connection
method like so.
# Rails method
ActiveRecord::Base.establish_connection({
adapter: 'informix',
database: 'dbname@servername', # servername as defined in sqlhosts
username: 'username',
password: 'password'
})
# Plain old ruby with informix gem
require 'informix'
db = Informix.connect('dbname@servername', 'username', 'password')
Summary
- Install Informix Client SDK
- Create and configure $INFORMIXDIR/etc/sqlhosts file
- Ensure environment variables are set for
$INFORMIXDIR
and$INFORMIXSERVER
- Install informix gem or active record adapter and configure Rails/Ruby